How to setup Pinia in Nuxt 3
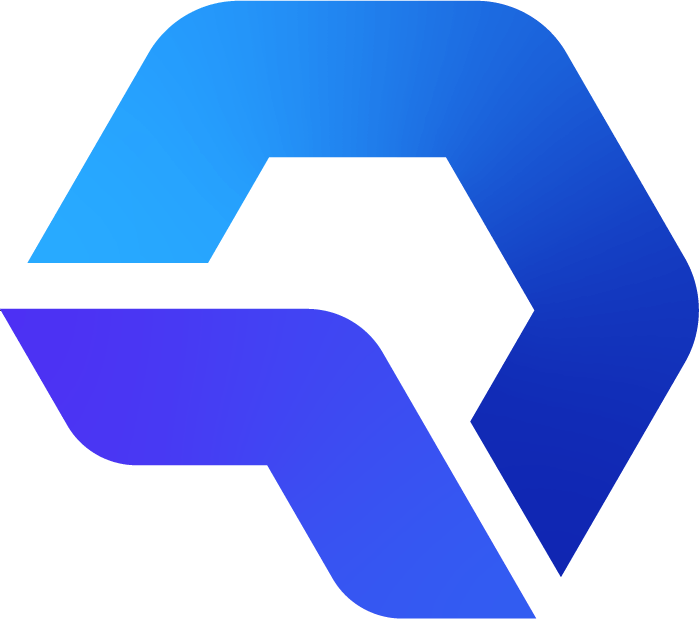
insidewebdev
Last updated:
Table of Contents
Set up
npm i @pinia/nuxt pinia
Add Pinia to your nuxt.config file
nuxt.config.ts
export default defineNuxtConfig({
buildModules: ['@pinia/nuxt'],
})
Create a store module
Create a folder called stores
and then add your store there , in this case auth.ts
:
stores/auth.ts
import { defineStore } from 'pinia'
export const useAuthStore = defineStore('auth', {
state: () => ({
user: null,
}),
getters: {
isLoggedIn: (state) => !!state.user
},
actions: {
async initAuth() {
let user = this.user
if (!user) {
const response = await $fetch('/api/user')
user = response.user
}
this.updateUser(user)
},
async login (payload) {
// some login logic
},
async register (payload) {
// some register logic
},
async logOut() {
this.updateUser(null)
},
updateUser(payload) {
this.user = payload
}
},
})
This is how a basic store that handles the authentication would like.
Use the module.
Now let's use the newly created store module in our vue component
<template>
<div>{{ isLoggedIn }}</div>
</template>
<script setup>
import { useAuthStore } from "~~/stores/auth";
const authStore = useAuthStore();
// Accessing getters and state
const { user, isLoggedIn } = storeToRefs(authStore);
await authStore.initAuth();
</script>
Conclusion
It's super easy to install and use Pinia in Nuxt 3.
Pinia is the next generation of store management for Vue, and I personally use it over Vuex