How to build your first REST API using express
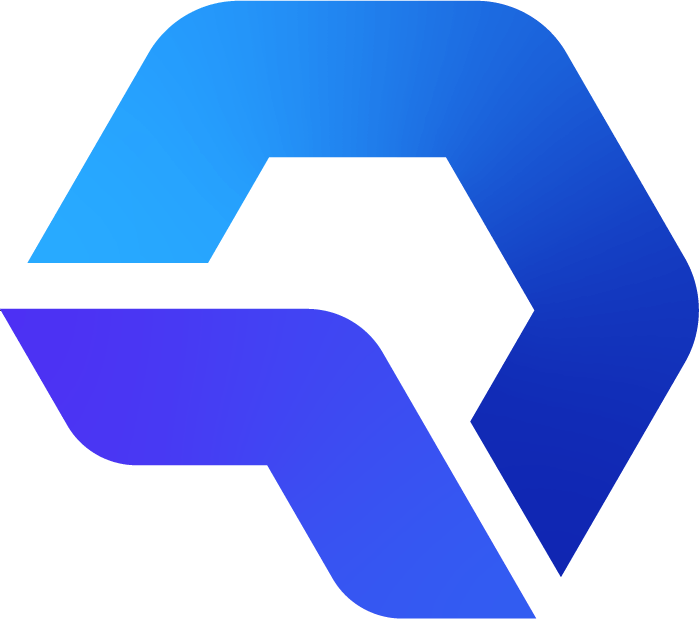
insidewebdev
Last updated:
Express has become one of the most popular nodejs frameworks and nowdays it's a framework that a lot of developers choose to build their apps.
So let's build a express restful api with some basic CRUD operations
Table of Contents
You can find the video format of this article on this video:
Prerequisites
First of course we will need nodejs
Install it using brew
brew install node
or alternatively through their official website nodejs.org
Setup
Let's start by creating a new folder
mkdir express_api
and cd into it
cd express_api
then init a new npm project
npm init -y
now will have a package.json
file created
It's important to add type
as module
, otherwise we won't be able to import and export modules using the new syntax.
{
...
"author": "",
"type": "module",
"license": "ISC",
...
}
now we can install express
, body-parser
and uuid
npm install express body-parser uuid
and also nodemon
as a dev dependency
npm install -D nodemon
Creating the server
Create a index.js
file in the root directory and add the following
import express from "express"
import bodyParser from "body-parser"
const app = express()
app.use(bodyParser.json())
app.listen(3000, function() {
console.log('Hey server is running on port 3000');
})
now if you go to http://localhost:3000/
should be able to see a cannot GET message
Congrats you just made your fist NodeJs server using express 🎊
Adding your first route
Adding a route is as simple as adding the following code
app.use(bodyParser.json())
app.get('/hello', function (req, res) {
res.send('world')
})
app.listen(3000, function() {
console.log('Hey server is running on port 3000');
})
now if you go to http://localhost:3000/hello
you should see world
returned
Routers
Now that we've seen the basics let's start moving our routes from the index.js
file to one called users.js
inside a folder called routes
The name of the file is users since we are going to be creating CRUD operations with the model user
import express from "express"
import { getUsers, createUser, deleteUser, getOneUser, updateUser} from "../controllers/user.js"
const router = express.Router()
router.get('/users', getUsers)
router.post('/users', createUser)
router.get('/users/:id', getOneUser)
router.delete('/users/:id',deleteUser)
router.put('/users/:id', updateUser)
export default router
as you can see we are importing the actual operations from the controllers
import express from "express"
import { getUsers, createUser, deleteUser, getOneUser, updateUser} from "../controllers/user.js"
const router = express.Router()
Let's go back to the index.js
file and add this line of code importing the newly created router
import userRouter from "./routes/user.js"
app.use(bodyParser.json())
app.use('/api', userRouter)
app.listen(3000, function() {
console.log('Hey server is running on port 3000');
})
Controllers
Inside the controllers is where all the magic happens
Create a file called user.js
inside a folder called controllers
and add the following code
import {v4 as uuidV4} from "uuid"
let users = [
{
name: 'jhon',
age: 20,
id: uuidV4()
},
{
name: 'amanda',
age: 22,
id: uuidV4()
},
{
name: 'rick',
age: 120,
id: uuidV4()
}
]
export const getUsers = function(req, res) {
res.json(users)
}
export const createUser = function(req, res) {
const { name, age } = req.body
users.push({
name,
age,
id: uuidV4()
})
res.json(users)
}
export const getOneUser = function(req, res) {
const userId = req.params.id
const user = users.find(function(user) {
return user.id === userId
})
res.json(user)
}
export const deleteUser = function(req, res) {
const userId = req.params.id
users = users.filter(function(user){
return user.id !== userId
})
res.json(users)
}
export const updateUser = function(req, res) {
const userId = req.params.id
const {age, name} = req.body
users = users.map(function(user) {
if(user.id === userId) {
return {
name,
age,
id: user.id
}
}
return user
})
res.json(users)
}
Note that instate of using a database we are using an array
let users = [
{
name: 'jhon',
age: 20,
id: uuidV4(),
},
{
name: 'amanda',
age: 22,
id: uuidV4(),
},
{
name: 'rick',
age: 120,
id: uuidV4(),
},
]
this will do the trick for now
Testing the API
You can use a tool like insomnia or postman both are free tools and work well
Get all users
Making a [GET]
to /api/users
will return a list of all the users
[
{
"name": "jhon",
"age": 20,
"id": "4e2cdbf4-a807-4a16-a4d2-5334c35159cf"
},
{
"name": "amanda",
"age": 22,
"id": "5623c2f4-77f3-4586-b7cf-a807417110e7"
},
{
"name": "rick",
"age": 120,
"id": "2427ee03-1c17-4454-b19f-180252651669"
}
]
Get one user
Making a [GET]
to /api/users/{user-id}
will return that user
{
"name": "jhon",
"age": 20,
"id": "4e2cdbf4-a807-4a16-a4d2-5334c35159cf"
}
Create one user
Making a [POST]
to /api/users
with the following body will return the updated list with the new user on it
{
"name": "amanda",
"age": 22
}
[
{
"name": "amanda",
"age": 22,
"id": "5623c2f4-77f3-4586-b7cf-a807417110e7"
},
{
"name": "rick",
"age": 120,
"id": "2427ee03-1c17-4454-b19f-180252651669"
}
]
Update one user
Making a [PUT]
to /api/users/{user-id}
with the following body will return the updated list with the updated user on it
{
"name": "amanda",
"age": 22
}
[
{
"name": "amanda",
"age": 22,
"id": "5623c2f4-77f3-4586-b7cf-a807417110e7"
},
{
"name": "rick",
"age": 120,
"id": "2427ee03-1c17-4454-b19f-180252651669"
}
]
Delete one user
Making a [DELETE]
to /api/users/{user-id}
will return the new list without the deleted user
[
{
"name": "amanda",
"age": 22,
"id": "5623c2f4-77f3-4586-b7cf-a807417110e7"
},
{
"name": "rick",
"age": 120,
"id": "2427ee03-1c17-4454-b19f-180252651669"
}
]
Wrapping up
We've seen how easy it was creating an API using express and that's why developers love using express.
All the code can be found on this repository